There's something poetic about translating the age-old game of chess into Python. The journey began with a simple dream, a desire to blend the traditional with the modern, to merge the time-honored logic of chess with the innovative spirit of programming. And where better to start than with the battlefield itself: the board.
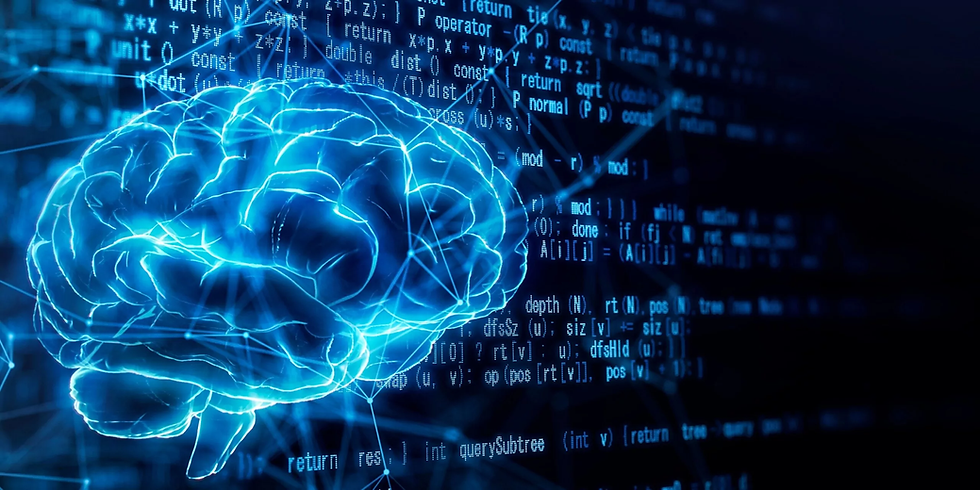
Using Python's versatile list comprehensions, I breathed life into the 8x8 square arena:
"The line self.grid = [[None for _ in range(8)] for _ in range(8)] might seem simple, but it's the heart of the game, the foundation awaiting its warriors."
Each chess piece, from the humble pawn to the majestic king, was given a class. Each class inherited from the overarching ChessPiece, ensuring a harmonious blend of efficiency and clarity. The King, for instance, was brought to life with elegance:
"Through class King(ChessPiece):, the regal piece got its digital identity, complete with attributes like its position (x, y) and color."
Example: King
import pygame
# Here I am creating a base class for all the chess pieces. This class will hold the common attributes and methods shared by all pieces.
class ChessPiece:
def __init__(self, x, y, color, board):
self.x = x
self.y = y
self.pos = (x, y)
self.board = board
self.color = color
def get_valid_moves(self):
"""To be overridden by subclasses with the actual logic to get valid moves for this piece."""
pass
# Moving on to create individual classes for each type of chess piece. Each class will define its own unique set of valid moves.
class King(ChessPiece):
def __init__(self, x, y, color, board):
super().__init__(x, y, color, board)
# I'll use 'K' to represent a king in the notation
self.notation = 'K'
def get_valid_moves(self):
# Here, I need to implement the logic to get all valid moves for a king
pass
# Next, I'll create classes for the Queen, Rook, Bishop, Knight, and Pawn, each overriding the get_valid_moves method to define their valid moves.
# Now, I am defining the ChessBoard class to represent the chessboard and handle the setup of the initial configuration.
class ChessBoard:
def __init__(self, tile_width, tile_height, board_size):
# ... (existing initialization code)
# Setting up the initial configuration with the standard setup for a chess game
self.config = [
['rR', 'rN', 'rB', 'rQ', 'rK', 'rB', 'rN', 'rR'],
['rP', 'rP', 'rP', 'rP', 'rP', 'rP', 'rP', 'rP'],
# ... (remaining rows for the initial configuration)
]
def _setup(self):
for y_ind, row in enumerate(self.config):
for x_ind, x in enumerate(row):
tile = self.get_tile_from_pos((x_ind, y_ind))
if x != '':
color, piece = x[0], x[1]
color = 'red' if color == 'r' else 'black'
# Here, I am creating the appropriate chess piece based on the notation in the config
piece_class = {'K': King, 'Q': Queen, 'R': Rook, 'B': Bishop, 'N': Knight, 'P': Pawn}
tile.occupying_piece = piece_class[piece](x_ind, y_ind, color, self)
# ... (Other parts of your existing code)
The challenge of implementing the intricate dance of chess moves was a nostalgic trip, reminding me of days spent over the board, plotting and strategizing.
Comments